decadron New Recruit 25 Posts user info edit post |
Here's a program I've started writing that represents the natural numbers in an interesting way, looking for a pattern in the relationship between the prime numbers. I talked to Dr. Chabay about it, and while she has been immensely helpful I thought I would post here to see if I could get even more help. Here is an excerpt of our correspondence that explains the concept:
While bored at a conference one of the attendees was doodling by writing the naturals in a spiral pattern:
6 <-- 5 <-- 4 | ^ | | 7 2 --> 3 | | 8 --> 9 --> 10
he noticed that prime numbers apparently always lay diagonally to each other when arrayed in this fashion. To explore this further I want to write a program that would display several of these spirals overlain over each other, incrementing the natural number at the center, creating a cube of n^3 spheres (each representing a natural number), colored according to their compositeness or primeness. Then the program should draw diagonals betwen primes, both within a spiral and between the layered spirals of the cube. For example the second 'layer' of this cube might start at 3: 7 <-- 6 <-- 5 | ^ | | 8 3 --> 4 | | 9 --> 10 --> 11
So hopefully that explains the concept decently. Here is my code so far, I tried to streamline it for this post. Sorry, I haven't documented this code very well at all.
from visual import * import math
def primes(n): # a prime list generator used by the isprime function below. if n==2: return [2] elif n<2: return [] s=range(3,n+2,2) mroot = n ** 0.5 half=(n+1)/2 i=0 m=3 while m <= mroot: if s[i]: j=(m*m-3)/2 s[j]=0 while j<half: if j >= len(s): break s[j]=0 j+=m i=i+1 m=2*i+3 return [2]+[x for x in s if x]
def isprime(aNumber): '''return True if the number is prime, false otherwise''' if aNumber < 2: return False if aNumber == 2: return True if (( aNumber / 2 ) * 2 == aNumber) : return False else: klist = primes(int(math.sqrt(aNumber+1))) for k in klist[1:]: if (( aNumber / k ) * k == aNumber ): return False return True
def redprime(p): # a function I wrote for use in coloring primes red and composites blue. if isprime(p): return color.red else: return color.blue
## User determined ####### edge = 5 # the size of the cube center = 4 # the natural number at the center of the bottom square ####### #Inits i = 1 j = 1 unitvec_x = vector(1,0,0) #used in the two while loops to unitvec_y = vector(0,1,0) #move the pointer sphere around the spiral unitvec_z = vector(0,0,1)
for z in range(edge): n = center + z L = -1 s = sphere(pos = (0,0,z), radius = .1, color = color.green) for d in range(edge): while j <= d: s.pos = s.pos + L*unitvec_x n = n + 1 s1 = sphere(pos = s.pos, radius = .1, color = redprime(n)) s1l = label(pos=s1.pos, xoffset=5, yoffset=5, color=color.white, text=str(n),box=0, opacity=0) j = j + 1 j = 1 while j <= d: s.pos = s.pos + L*unitvec_y n = n + 1 s1 = sphere(pos = s.pos, radius = .1, color = redprime(n)) s1l = label(pos=s1.pos, xoffset=5, yoffset=5, color=color.white, text=str(n),box=0, opacity=0) j = j + 1 j = 1 L = -L s.pos = (0,0,z) s.color = redprime(center + z) print "done" arrow(pos = (0,1,4), axis = (-1,-1,0), shaftwidth = .1, color = color.green) arrow(pos = (0,0,3), axis = (0,-1,-1), shaftwidth = .1, color = color.green)
What I need to do now, and what is quite intractible for me (having never used Python before) is figuring out how to draw the arrows between primes. So I can conceptualize two possibilities, either naming the spheres so that I can refer to them (and Dr Chabay says I could even give them a primeness attribute) or create a data structure, some kind of list or dictionary, that I could refer to to give the coordinates for arrows between neighboring primes. If you don't change the center variable I put two example arrows. Thanks in advance for any help you care to give on this project. 4/12/2006 7:52:52 PM
|
skokiaan All American 26447 Posts user info edit post |
use [ code ] tags and paste that shit in again so the spacing looks right
as I suspected -- math nerds were all over this shit a long time ago:
http://mathworld.wolfram.com/PrimeSpiral.html
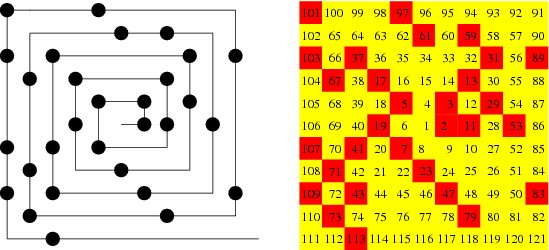
[Edited on April 12, 2006 at 8:15 PM. Reason : I see you are a freshman. The first step to solving any problem is to see if its been done already] 4/12/2006 8:10:37 PM
|
LimpyNuts All American 16859 Posts user info edit post |
all primes except 2 are odd numbers. since odd numbers are diagonal to each other when arrayed in this way, prime numbers can only be diagonal to adjacent prime numbers.
[Edited on April 13, 2006 at 11:30 AM. Reason : ] 4/13/2006 11:19:24 AM
|
OmarBadu zidik 25067 Posts user info edit post |
you are a complete idiot 4/13/2006 11:27:06 AM
|
LimpyNuts All American 16859 Posts user info edit post |
^me?
by calculating the primes while you generate the spiral you save yourself the CPU cycles used to search the array of primes for each number. the larger the spiral, the more CPU cycles that saves.
my other option of preloading an array like:
isprime[1] = false isprime[2] = true isprime[3] = true isprime[4] = fase ...
saves you those same CPU cycles once again because you don't have to search the array for every number you add to the spiral.
explain to me why that makes me an idiot 4/13/2006 11:42:31 AM
|
OmarBadu zidik 25067 Posts user info edit post |
no limpy - you are bright - the thread originator is a retard 4/13/2006 12:03:31 PM
|
spöokyjon ℵ 18617 Posts user info edit post |
I never realized all prime numbers >2 were odd!!! 4/13/2006 1:15:37 PM
|
LimpyNuts All American 16859 Posts user info edit post |
the mathworld article is just as bad:
Quote : | "Unexpected patterns of diagonal lines are apparent in such a plot" |
wtf? you could randomly highlight any set of odd numbers in that arrangement and patterns of diagonal lines would appear. 4/13/2006 1:41:39 PM
|
decadron New Recruit 25 Posts user info edit post |
Thanks ya'll, esp skokiaan for the mathworld link. And thanks Limpynuts for the tips on saving CPU cycles, though I mostly just want to get the inter-spiral connections drawn and not worry about resource usage. Yes, Stanislaw Ulam did extensive work on this pet project of his, going so far as to get time on the Maniac supercomputer to generate a very large spiral. I must not have explained very clearly the difference between what I am attempting to do. As I understand, Ulam's research into these spirals didn't turn up any results. This VPython project would create several such spirals, each beginning at a different natural number, and layer them, looking for relationships between the spirals. Also, I should note that the spiral centered at 3 has two very interesting diagonals. I constructed this by hand up to about 250, and noticed that on one of the diagonals containing 3 all the nonprimes were divisible by 3. The parallel diagonal containing 5 exhibited similar behavior, with each composite being divisible by 5, though extending the spiral broke this psuedopattern. So I must be missing something, and perhaps someone could communicate what is so obvious to you (not surprising, that's why I posted here, thinking that those who frequent this board could be exxpected to have insight into this problem) to me with more understanding, class and skill. Does the triviality of odds and evens forming all diagonals persist in hexagonal spirals or if each layer of what I described originally was centered at a prime number? 4/14/2006 8:36:14 PM
|
skokiaan All American 26447 Posts user info edit post |
It sounds like mathematicians have looked at this as an oddity but have not really found any regular patter. If you really want to continue on your train of thought, I would suggest thinking about a generalized algorithm for n-sided spirals in n layers and/or dimensions.
Also, I would try to figure out a better way to find diagonals/correlation than visual inspection. Primes are one of the most heavily researched things. I'm sure there is literature out there 4/14/2006 10:17:57 PM
|
Lowjack All American 10491 Posts user info edit post |
http://www.claymath.org/millennium/Riemann_Hypothesis/
Quote : | "Riemann Hypothesis Some numbers have the special property that they cannot be expressed as the product of two smaller numbers, e.g., 2, 3, 5, 7, etc. Such numbers are called prime numbers, and they play an important role, both in pure mathematics and its applications. The distribution of such prime numbers among all natural numbers does not follow any regular pattern, however the German mathematician G.F.B. Riemann (1826 - 1866) observed that the frequency of prime numbers is very closely related to the behavior of an elaborate function
?(s) = 1 + 1/2s + 1/3s + 1/4s + ...
called the Riemann Zeta function. The Riemann hypothesis asserts that all interesting solutions of the equation
?(s) = 0
lie on a certain vertical straight line. This has been checked for the first 1,500,000,000 solutions. A proof that it is true for every interesting solution would shed light on many of the mysteries surrounding the distribution of prime numbers." |
[Edited on April 15, 2006 at 4:09 PM. Reason : 465] 4/15/2006 4:08:54 PM
|
BigMan157 no u 103352 Posts user info edit post |
^that's what i thought of too 4/15/2006 4:26:11 PM
|
kiljadn All American 44689 Posts user info edit post |
PYTHON IS NOT THAT GOOD. I PREFER TO USE BOACONSTRICTOR 6.7 WITH VIPER 3.67 4/17/2006 11:03:03 AM
|
LimpyNuts All American 16859 Posts user info edit post |
Quote : | "Yes, Stanislaw Ulam did extensive work on this pet project of his, going so far as to get time on the Maniac supercomputer to generate a very large spiral." |
I don't think you realize how many CPU cycles you are wasting in how you handle primality testing. If you're going to run something on a supercomputer you want it to be as efficient as possible to maximize what you get out of it in the time you're alloted.
Using the sieve of eratosthenes to generate the primes list while you make the spiral would not require you to store the primes less than the number you are currently evaluating in memory.
A slightly less efficient way to do it would be to populate an array like I said before: IsPrime[1] = False, IsPrime[2] = True, etc. instead of creating a list of primes. This can be done by modifying your prime list generator a little bit. When you determine a number is prime, instead of adding it to an array, set IsPrime[index_of_prime] to True. That way IsPrime[578639] would take only a small fraction of the number of CPU cycles that calling a function to search a list of primes for 578,639 would.
It is a very small code change for a very big performance impact. 4/17/2006 5:28:43 PM
|
Incognegro Suspended 4172 Posts user info edit post |
You know what's funny... you acting like you know anything about optimization, making all these dumb ass suggestions (like the sieve, which is virtually useless in non-trivial primality computations due to its obscene memory overhead), when the FIRST FUCKING THING OUT OF YOUR MOUTH if you wanted to help him optimize his approach should have been:
DO NOT WRITE THIS IN AN INTERPRETED LANGUAGE
No pattern in primality observed within the range of a 32-bit int will be considered academically meaningful. People were crunching Mersenne primes waaay out of this range before they had so much as pocket calculators. I'm pretty fucking arrogant and let me tell you that it's never once occured to me to investigate primality. Number theory is perhaps the most beaten dead horse in any rational discipline. You're not going to find anything interesting without a serious fucking supercomputer, and you're not even going to be allowed to view a serious supercomputer from a distance if they catch wind of the fact that you're writing this in Python.
[Edited on April 18, 2006 at 12:47 AM. Reason : I really tried to ignore this thread, but I just couldn't anymore] 4/18/2006 12:38:38 AM
|
skokiaan All American 26447 Posts user info edit post |
A black person trying to tell a white person about something other than basketball, watermelon, and dancing? The world's flipped upside down.
You might find this hard to believe, but people learn things by studying things that other people have already studied.
FYI, the fastest optimization is to just use the primes that people have already calculated. 4/18/2006 12:53:04 AM
|
Incognegro Suspended 4172 Posts user info edit post |
I'm not black, dumbass. 4/18/2006 12:57:53 AM
|
skokiaan All American 26447 Posts user info edit post |
The lady doth protest too much, methinks 4/18/2006 1:02:25 AM
|
Shadowrunner All American 18332 Posts user info edit post |
Quote : | "Also, I should note that the spiral centered at 3 has two very interesting diagonals. I constructed this by hand up to about 250, and noticed that on one of the diagonals containing 3 all the nonprimes were divisible by 3. The parallel diagonal containing 5 exhibited similar behavior, with each composite being divisible by 5, though extending the spiral broke this psuedopattern. " |
So rather than looking it as a spiral, make algebraic notations for your diagonals and then work in modular arithmetic. I'll make it easy for you to start, since the work is trivial.
With a spiral centered at 3 and oriented in the way you've indicated (so that in the first 2x2 square, the 3 is the lower-left corner), and letting k be the distance away from 3 you're interested in, the 4 numbers on the diagonal at a distance of [i]k[/k] are expressed thusly:
3 + 4k^2 3 + 4k^2 - 2k 3 + 4k^2 + 2k 3 + 4k^2 + 4k
Using those expressions, it's very easy to calculate the diagonals mod 3 for any value of k (mod 3). Obviously, if k is divisible by 3, all the diagonals will be likewise divisible by 3, as I'm sure you've noticed. So your homework is to look at k=1(mod 3) and k=-1(mod 3) and the resulting diagonals, to show why the diagonals indivisible by 3 must be prime. Or rather, where any such proof breaks down. Even though finding a counterexample is all you need to do, you'll get more out of the exercise to see why the counterexample exists than to actually find it. You'll also find that you were pretty close to mapping out by hand to the point where the first counterexample on the second diagonal falls (k=7 for one diagonal, and 8 for the other).
Bonus points for showing why a proof for hexagonal spirals (and other n-spirals in general) will also fail, regardless of whether your starting number is prime or not. In addition, I believe it may be possible to construct a general expression for a counterexample on n-spirals given any starting number (related to why the proofs won't work), but I didn't bother doing the construction.]] 4/18/2006 2:13:51 AM
|
Shadowrunner All American 18332 Posts user info edit post |
And here I was hoping for a good math thread.  4/20/2006 10:07:28 PM
|
|